이번 포스팅은 Flask + uWSGI + Nginx를 연결하여
웹 애플리케이션을 배포하는 포스팅입니다.
Flask ( 웹 애플리케이션 )
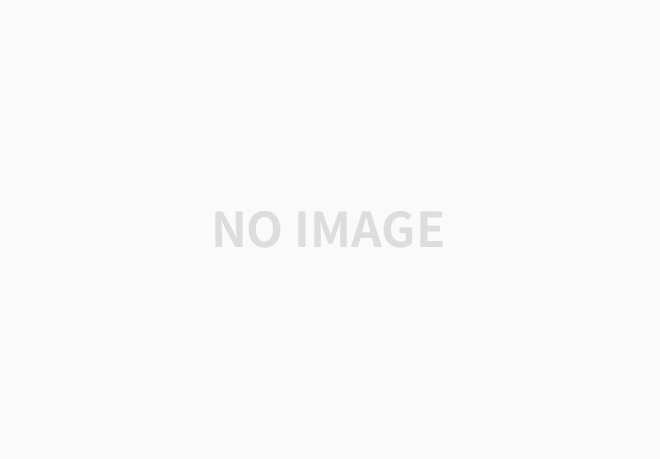
Flask는 파이썬으로 작성된 마이크로 웹 프레임워크 중 하나이다.
Flask 설치
$ pip install flask
> pip를 사용하여 간단하게 플라스크를 설치한다.
Flask 기반 웹 어플리케이션 작성
Flask 기반 웹 어플리케이션을 작성한다.
우선은 구동을 위해 간단하게 작성 진행, 파일명은 application.py 로 생성 (아래 wsgi.py 파일에서 사용)
from flask import Flask
app = Flask(__name__)
def index():
return "Hello Flask"
if __name__ == '__main__':
app.run()
uWSGI ( 통신을 위한 프로토콜 )
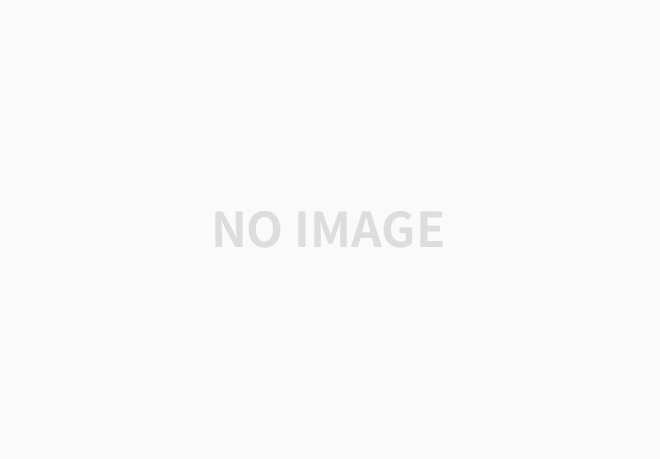
uWSGI가 nginx와 Flask 앱 중간에서 미들웨어 역할을 하게 된다.
WSGI는 Web Server Gateway Interface의 약어로 웹서버와 웹 애플리케이션이 어떤 방식으로 통신하는가에 관한 인터페이스로써, 웹 서버와 웹어플리케이션 간의 소통을 정의해 애플리케이션과 서버가 독립적으로 운영될 수 있게 돕는다
uwsgi 설치
# uWSGI
$ apt-get install uwsgi
# python과 uWSGI를 연결하는 플러그인
$ apt-get install uwsgi-plugin-python3
> uwsgi를 설치하고, python과 uwsgi를 연결하는 플러그인을 설치한다.
> uwsgi-plugin-python3이 아닌 uwsgi-plugin-python으로 설치하게 되면, 실제 파이썬이 실행시 파이썬 2.*버전으로 작동된다. 꼭 맞는 버전의 플러그인을 설치해야함
> 실수로 uwsgi-plugin-python를 설치할경우, 지우고 새로 설치해야함
$ apt-get remove uwsgi-plugin-python
# 위와 같이 지우고 새로 설치
wsgi.py 파일 생성
기존 플라스크 앱 메인 파일 외에 uWSGI 서버 실행을 위한 모듈을 생성.
$ vi [프로젝트경로]/wsgi.py
from application import app
if __name__ == "__main__":
app.run()
> uWSGI를 이용하여 서버에 구동하기 위해, 우선 작성한 어플리케이션과 uwsgi를 연결시켜주기 위한 wsgi.py 파일 생성
> from 에는 플라스크로 생성한 프로젝트 이름(application.py)과 import는 해당 프로젝트에서 플라스크를 생성한 애플리케이션(app) 이름을 넣는다.
uwsgi 설정 파일
/etc/uwsgi/app-available 폴더에 uwsgi.ini이라는 설정 파일을 생성한다.
$ vi /etc/uwsgi/apps-available/uwsgi.ini
[uwsgi]
chdir = [프로젝트 경로]
callable = app [어플리케이션이름]
lugin = python3
module = wsgi [실행할파일이름]
master = true
socket = /tmp/uwsgi.sock [소켓파일명]
chmod-socket = 666 v
accuum = true d
ie-on-term = true
ignore-sigpipe=true i
gnore-write-errors=true
disable-write-exception=true
설정 파일 적용 명령어
# 처음 실행 시 (소켓생성)
$ sudo service uwsgi retart
# 설정사항 혹은 flask 웹 어플리케이션 소스 변경 후 적용
$ sudo service uwsgi reload
> service uwsgi restart : uwsgi 재시작 명령어 (소켓을 새로 생성해야 하는 경우는 재시작을 해야 함)
> service uwsgi reload : 설정 파일 변경 후 적용 / flask 웹 애플리케이션 코드 변경 적용
심볼릭 링크 설정
$ ln -s /etc/uwsgi/apps-available/uwsgi.ini /etc/uwsgi/apps-enabled/
Nginx (웹서버)
Nginx는 apache와 같은 웹서버로, 차이점은 nginx는 비동기 이벤트 기반으로 만들어진 점이다.
apache에 비해 적은 메모리를 사용하는 장점이 있음
Nginx 설치
$ apt-get install nginx
Nginx 설정 파일
/etc/nginx/sites-available 폴더 아래에 설정 파일 생성
$ vi /etc/nginx/sites-available/[설정파일명]
server {
listen 80;
server_name [도메인];
root [프로젝트폴더];
location / {
try_files $uri @app;
access_log off;
}
location /favicon.ico {
deny all;
log_not_found off;
access_log off;
}
location @app {
access_log /var/log/nginx/access.log;
include uwsgi_params;
uwsgi_pass unix:/tmp/uwsgi.sock;
uwsgi_max_temp_file_size 20480m;
uwsgi_buffering off;
uwsgi_ignore_client_abort on;
uwsgi_buffers 2560 160k;
uwsgi_buffer_size 2560k;
uwsgi_connect_timeout 30s;
uwsgi_send_timeout 30s;
uwsgi_read_timeout 30s;
uwsgi_busy_buffers_size 2560k;
uwsgi_temp_file_write_size 2560k;
proxy_read_timeout 30s;
proxy_connect_timeout 75s;
}
}
> 도메인 없이 localhost에서 테스트할 경우에는 server_name를 localhost로 지정
심볼릭 링크 생성
$ ln -s /etc/nginx/sites-available/[설정파일명] /etc/nginx/sites-enabled
> 절대 경로로 선언해야 함.
> 심볼릭 링크를 연결해야 사이트가 활성화된다
Nginx 재시작
# nginx 설정파일 문법오류 체크
$ nginx -t
# 위에서 success가 되었다면
$ service nginx restart
> uWSGI 소켓 연결 전이라면, 서버 접속 시 502 Bad Gateway 가 뜬다.
Nginx + uWSGI + Flask 전체 프로세스
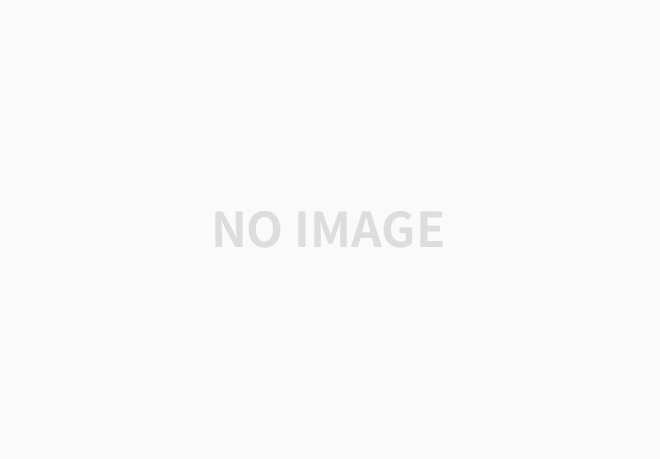
> 클라이언트가 요청을 보내면, 가장 먼저 Nginx가 받는다.
> 그리고 unix 소켓을 통해 nginx와 uwsgi를 연결한다.
: nginx 설정 파일의 uwsgi_pass unix:/tmp/uwsgi.sock;
: uwsgi 설정파일의 socket = /tmp/uwsgi.sock
> 위의 각 설정 파일 안의 소켓을 통해 연결된다.
> nginx와 uwsgi를 socket으로 연결해서 flask 앱이 실행되는 구조
'Python Web Framework > Django, Flask' 카테고리의 다른 글
[Golang + MongoDB] 몽고+고랭으로 CRUD 구현하기 (1) | 2020.06.10 |
---|---|
[Django MySQL] Python으로 DB에 접속하기 (0) | 2020.02.08 |
[Django] Trouble Shooting (0) | 2020.01.24 |
[Nginx + Gunicorn + Django] API 서버 구축 (0) | 2020.01.20 |
Django_Admin (0) | 2019.04.08 |